The following topics will be discussed in this blog:
Input Variable
You can define particular input variables to act as module parameters in Terraform, just like you do with bash scripting and PowerShell, letting you modify the attributes of a module without affecting its source code.
resource "local_file" "var_example" {
name = var.name
title = var.title
}
It’s time to start defining your variables. You’ll need to add a variable block to the file to accomplish this.
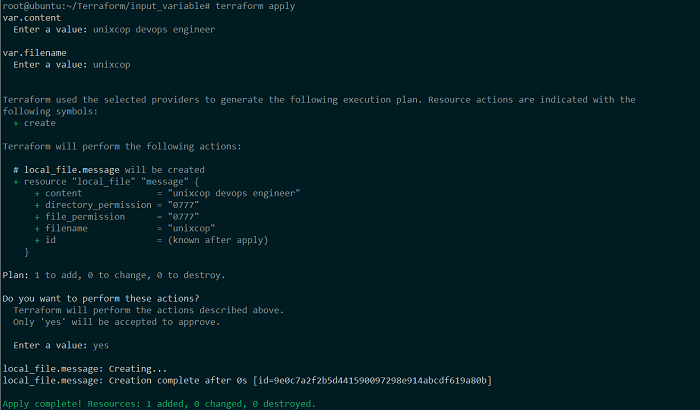

The following optional properties will be present in the variable block:
Type; Determines what type of variable is being utilized. The execution will be paused when the specified value doesn’t match the type, and you will receive the following errors:
Error: Invalid value for input variable
The value entered for variable "{name of the variable}" is not valid: a {type of the variable} is required.
Default; A variable’s default value is specified. We would be asked to enter values for each variable when the variable does not exist and the value is not provided.
Description; A brief explanation of the variable as well as its function.
Validation; is a block that lets you create your own validation rules.
Sensitive; When set to true, it protects data from being accidentally accessible in CLI and log output.
variable "variable-name-Example" {
type = string|bool|list|...
default = "enter the default value"
description = "variable-description"
validation {...}
sensitive = true|false
}
In the following scenario, I used a mixture of the described variables to define numerous variables.
variable "content_example" {
}
variable "pnfn" {
type = number
description = "The filename is defined by the file number."
}
variable "psfn" {
type = string
default = "The name of a file in part."
}
variable "psfnSensitive" {
type = string
default = "file"
description = "But it's a sensitive filename but its sensitive."
sensitive = true
}
variable "extension" {
type = string
description = "The extension of the file. Accepted file types are txt and jpeg."
validation {
condition = anytrue([var.extension == "txt", var.extension == "jpg"])
error_message = "Extension isn't working! Change the file type to txt or jpeg."
}
}
resource "local_file" "var_example" {
filename = "/root/Terraform/local_file_with_external_variable/${var.pnfn}-${var.psfn}-${var.psfnSensitive}.${var.extension}"
content = var.content_example
}
Let’s look at each variable individually.
Content: There are no attributes in this definition. As a result, if I don’t define its value inside a config file, I’ll be asked to do so later. It also has no specified validation or structure, thus any value from a string as a single character or as complicated as JSON will be accepted.
pnfn and psfn: These two variables have the same concept. The sole difference is that the type attribute on pnfn is set to a string, whereas the type attribute on psfn is set to a number. As a result, pnfn only accepts string values, whereas psfn will accept any integer.
psfn sensitive: This variable is identical to psfn but as well as it allows the string attributes. As a result, when the action plan is prompted, the string will obscure its value (sensitive).
Extension: To limit the extensions a user is permitted to create and add a confirmation block to verify the value is equal to text or png. Otherwise, an error will be thrown, the execution will be halted, and the error message will be displayed.
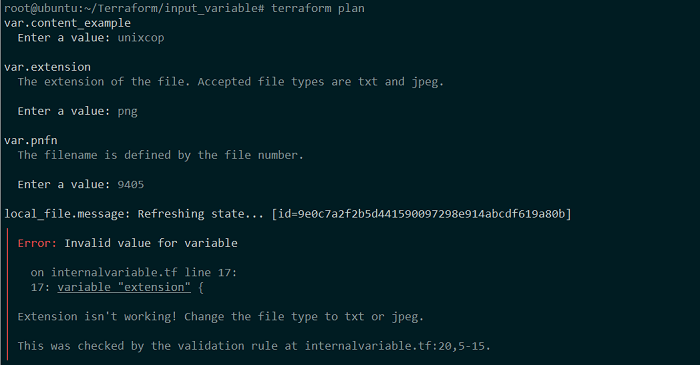
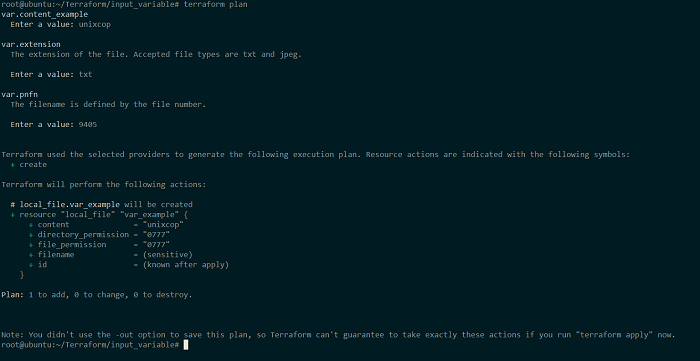
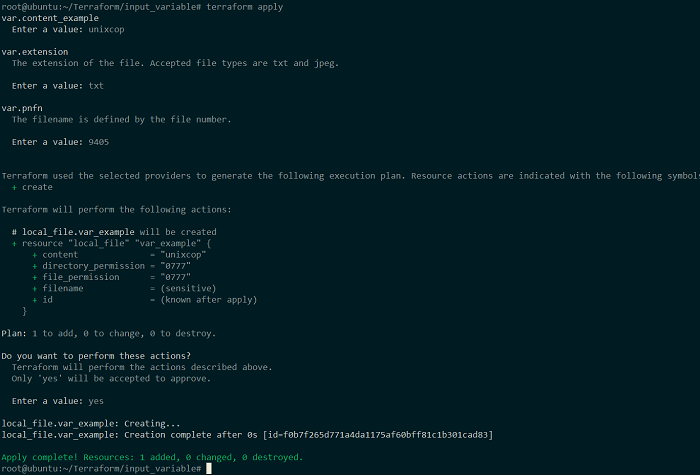
I ran the apply command 2 – 3 times to give an overview of these attributes. It failed the first time because I used a string rather than a number for the variable pnfn. It failed the second time because I changed the variable extension to anything other than text or png. The previous execution was completed successfully, prompting the execution plan with psfn concealed.
Types of input variables
I specified my variables as an integer or string with any value in the preceding example. There are, however, numerous additional types. Terraform currently accepts these value types:
String: A single alphanumeric value is represented as a string.
variable "string_example" {
type = string
default = "Unixcop"
}
Number: Numbers can be positive or negative.
variable "string_number_example" {
type = number
default = 8089405
}
Boolean: True or false value in a Boolean (Bool) expression.
variable "string_boolean_example" {
type = bool
default = true
}
List: A numerical arrangement of values that are the same is referred to as a list. Each element has a unique index that begins at zero. This type can be thought of as an array if you’re experienced with programming languages such as C sharp or Python.
variable "string_list_example" {
type = list
default = ["unixcop", "unixcop1", "unixcop2"]
}
Set: A numerical arrangement of values that are the same that contains no repetitions element.
variable "string_set_example" {
type = set
default = ["red", "blue", "yellow"]
}
Map: It’s a collection of data organized into key-value pairs.
variable "string_map_example" {
type = map
default = {
color = "blue"
hex = "#0000FF"
}
}
Tuple: A grouping of values of several types. Within the square brackets, the kind of variables to be utilized in a tuple is specified.
variable "string_tuple_example" {
type = tuple([string, bool, number])
default = ["unixcop", True, 9405]
}
Object: A complex data structure that can contain all of the previously mentioned variable kinds.
variable "string_tuple_example" {
type = object({
name = string
color = string
rollnumber = number
subjects= list(string)
})
default = {
name = "unixcop"
color = "blue"
rollnumber = 94
subjects= ["Math", "English", "Science"]
}
}
It’s also possible to mix and match type constraints, as shown below:
variable "string_list_example" {
type = list(number)
default = [91, 23, 5, 10, 54]
}
variable "string_set_example" {
type = set(string)
default = ["blue", "black", "red"]
}
Check this article for Input variable – Part2
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////